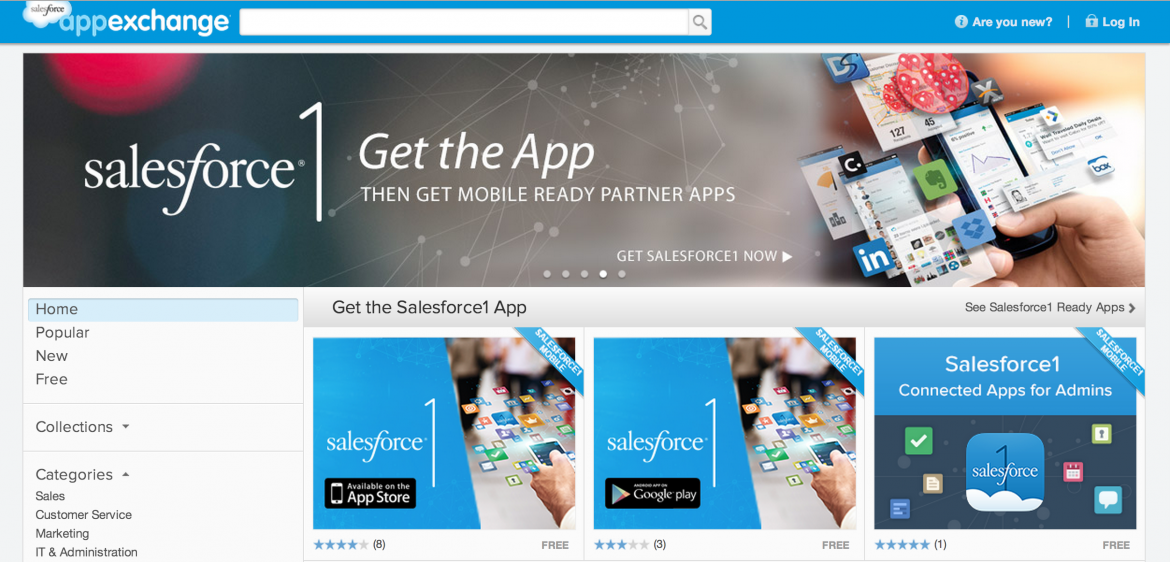
A common design challenge when building applications for the AppExchange is the need to load data right after the app has been installed.
Who might need this? A common use case is if your application uses custom objects instead of custom settings to store complex business logic related metadata. In order to automate the process of creating the data after every install and provide a seamless trial/installation experience, you might want to automatically create the data after the package finishes installing in your customer’s org.
Since managed packages don’t allow data to be packaged, typically this needs to be done as a part of the post-install script. If the dataset is small, you can hard code the data in to the script but this approach doesn’t scale to larger data sets.
One approach that has worked for us is to do the following:
- Export the data from the development org or another source as a JSON file
- Add the JSON file as a static resource in the managed package
- In the post-install script, load the data from the file and insert into Salesforce
Below is example code that illustrates this pattern:
[apex] global class Installer implements InstallHandler { global void onInstall(InstallContext context) { // If installing for the first time, setup data. if (context.previousVersion() == null) { // Example on how to load Static Resource containing JSON and to deserialize it and save as records. StaticResource someObjectStaticResource = [select Body from StaticResource where Name = 'Some_Object_Data']; List<Some_Object__c> someObjects = (List<Some_Object__c>) JSON.deserialize(someObjectStaticResource.Body.toString(), List<Some_Object__c>.class); insert someObjects ; } } } [/apex]
Enhancements:
- The script can be easily modified to use other data format types and parsers.
- For even larger data sets/files, you can package a batchable process to read chunks of the file at a time. Here’s a good example of a batchable process that uses a csv parser – http://developer.financialforce.com/customizations/importing-large-csv-files-via-batch-apex/
CREDIT – Thanks James Holmes for the sample code!